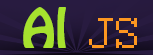
aljs
Classical Inheritance in JavaScript
``` Class.define('A', { _public: { init: function () { // if you define the constructor as protected or private it will simply not be // executed, that will not however prevent the class from being instantiated console.log('constructor in A'); this.parent(); }, a: function () { console.log('a in A'); }, b: function () { console.log('b in A'); }, c: 5 }, _protected: { p1: function () { console.log('p1 in A'); }, p2: function (message) { console.log(message); } }, listeners: { someEvent: function () { // Since event listeners are technically not methods of a class, // the private "parent" and "callParent" methods are not available here, // just like any other private and protected members. // The variable "this" does however reference the class instance. console.log('someEvent fired in A'); } } });
Class.define('B', { extend: 'A', _public: { init: function () { console.log('constructor in B'); this.parent(); }, a: function () { console.log('a in B'); }, b: function () { console.log('b in B'); this.p1(); this.p2(); this.p3(); }, getTreasure: function () { this.hiddenTreasure(); } }, _protected: { p1: function () { console.log('p1 in B'); // this.parent automatically sends the same arguments to the super method as were passed here, // unless you override them this.parent(); }, p2: function () { console.log('p2 in B'); this.callParent('p2', 'super class named method called'); }, p3: function () { console.log('p3 in B'); } }, _private: { hiddenTreasure: function () { console.log("you've found it!"); } }, listeners: { someEvent: function () { console.log('someEvent fired in B'); }, someOtherEvent: function () { console.log('someOtherEvent fired in B'); } } });
b = Class.create('B'); b.b(); b.getTreasure(); b.fire('someEvent');
b.on('someOtherEvent', function () { console.log('someOtherEvent fired in global scope'); console.log(arguments); });
b.fire('someOtherEvent', 'you', 'can', 'pass', 'data', 'to', 'the', 'listeners');
// remove all event listeners for the specified event, // you can remove them all if you want, works similarly to jQuery.unbind b.un('someEvent');
b.on('oneMoreEvent', function () { // this event can only be triggered once, try it! console.log('oneMoreEvent fired in global scope'); }, true);
// and last but not least, a test to see if "b" is an instance of "B" console.log(Class.is('B', b));
// oh, and one more thing, just like in PHP public visibility is implied, // so the following two declarations are identical: // // Class.define('MyClass', { // _public: { // init: function () { // //I'm a public constructor // }, // method1: function () { // //I can be accessed from anywhere // } // }, // _protected: { // method2: function () { // //I can only be accessed from within an instance of MyClass and all its derivatives // } // }, // _private: { // method3: function () { // //I can only be accessed from within an instance of MyClass // } // } // }); // // Class.define('MyClass', { // init: function () { // //I'm a public constructor // }, // method1: function () { // //I can be accessed from anywhere // }, // _protected: { // ... // // }); ```
Disclaimer:
The provided code snippet was developed entirely by Eugene Kuzmenko, no external libraries or tools were used in the process.
This was never intended to be a practical implementation, but rather a prove-of-the-concept, to show that this was indeed possible with javascript.
Project Information
The project was created on Aug 17, 2013.
- License: MIT License
- 1 stars
- git-based source control
Labels:
JavaScript
CrossPlatform
Custom
Small
Visibility
Accessmodifier
Public
Protected
Private
Inheritance
OOP